3DS Android SDK
Last updated: March 26, 2025
Use our 3D Secure (3DS) Android SDK to seamlessly handle the end-to-end 3DS authentication flow.
With a single method call, the SDK handles:
- collecting required device data
- communicating with the customer's bank
- presenting 3DS challenges when required
When used alongside our non-hosted sessions authentication solution, the 3DS SDK allows you to authenticate within the payment authorization flow. Alternatively, you can use the authentication result to complete the payment manually at a later time.
Additionally, the SDK is fully EMVCo and PCI-compliant, ensuring interoperability with card issuers and secure handling of your customers' data.
Information
The SDK only collects data which your app already has permission to collect – the customer will never be prompted to grant new permissions. The device data collected is encrypted throughout the authentication process but is never stored, and the SDK does not retain any personal information about the customer.
3DS allows you and your payment provider to share additional data with the customer's bank. For example, the customer's device ID and payment history.
This data allows the bank to assess the risk of the transaction more accurately, and determine which flow the customer will be required to go through:
- frictionless flow
- challenge flow
If the customer's bank is satisfied with the data and trusts that the cardholder making the payment is genuine, the transaction will be authenticated without interrupting the customer.
The customer is only shown a brief Processing UI, with their card scheme's branding.

If the bank requires additional evidence to authenticate the cardholder, they will prompt the customer to verify their identity.
To do this, the bank may use any of the following methods:
- one-time password – the customer is sent a single-use password or code via text or email, which they must enter
- single-select or multi-select – the customer is asked a multiple-choice question, with one or more selections allowed
- out-of-band – the customer is asked to confirm the transaction through another channel, typically their banking app
- HTML – the bank explicitly defines the view that should be presented to the customer via HTML, which can be that of any of the previous methods, or a custom approach

Information
To provide a consistent experience across different devices and schemes, there are standard templates and rules that the 3DS challenge screens adhere to. However, there is some leeway around styling. Our SDK gives you control over the display of the native challenges in your app, while ensuring that the rules are followed.
Add the SDK as a project dependency.
Initialize the SDK.
Configure authentication parameters.
Handle authentication results.
Customize the 3DS Challenge UI.
Make sure you have a test account with Checkout.com. To use the SDK, you'll also need to have onboarded with our non-hosted sessions authentication solution.
You'll also need the following:
- a public key, to initialize the SDK and authenticate your payment request
- a secret key or OAuth 2.0 client credentials, to use as an authentication mechanism
To get started, download the 3DS SDK for Android from GitHub.
Information
You can subscribe to the GitHub repository to be notified about updates to the SDK.
The minimum requirements to use the Android SDK are:
- Kotlin 1.8.10 or higher
- JDK 17 or higher
- Gradle 8.0.2 or higher
- Android Gradle Plugin 8.0
- Android API level 21 (Android 5.0) or higher as a build target
Information
The SDK makes use of Java 8+ language features. To run on Android level 25 (Android 7) or lower, you must enable desugaring.
Declare the 3DS SDK as an application or library dependency in your build.gradle
file, using Maven Repository or GitHub Packages.
1// Option 1: add the following to your project’s top-level Gradle file2allprojects {3repositories {4mavenCentral()5}6}78// Option 2: add the following to your project’s Gradle settings file9dependencyResolutionManagement {10repositories {11mavenCentral()12}13}1415// Add the following to your application or library's Gradle file16dependencies {17implementation("com.checkout:checkout-sdk-3ds-android:3.x.x")18}
Initialize the SDK and set the:
- environment – for example,
production
orsandbox
- locale
- 3DS challenge UI configuration
1// Option 1: initialize with default configuration2val checkout3DS = Checkout3DSService(context)34// Option 2: initialize with custom configuration5val checkout3DS = Checkout3DSService(6context,7Environment.PRODUCTION,8Locale.UK,9uiCustomization,10Uri.parse("https://sampleAppName.com/3ds_authentication")11)
The SDK's Checkout3DSService
class provides the core functionality to perform payment authentication via the authenticate()
method.
You only need to initialize the class once for the lifetime of the application.
You must specify an app context
when you initialize the SDK. All other configuration options are optional.
You can use the getWarnings()
method to check for any security warnings when you initialize the SDK, or at any time after initialization.
The following table outlines the possible id
values returned by the getWarnings()
method:
Warning ID | Severity | Description |
---|---|---|
|
| The app is running on a jailbroken device |
|
| The SDK has been tempered with |
|
| The app is running within an emulator |
|
| A debugger is attached to the app |
|
| The app is running on an unsupported OS version |
|
| The |
|
| The |
Before you can begin authentication using the SDK, your back end must first request an authentication session using the Sessions API's
post
Request a session
endpoint. See our API reference for all required and optional fields.In your application code, create an instance of AuthenticationParameters
and populate it with the values returned in the Session API response.
1val authenticationParameters = AuthenticationParameters(2sessionId = "sessionId",3sessionSecret = "sessionSecret",4scheme = "scheme"5)
With the authentication information, the SDK will take care of:
- gathering the necessary device data
- sharing the information with the customer's bank
- if necessary, presenting a native challenge screen to the customer
The type of challenge presented to the customer depends on the configuration of the customer’s bank.
When the authentication process is completed after you call the authenticate()
method, the SDK will return the authentication results:
- if authentication was successful, the SDK will return a
AuthenticationCompleted
object – you can then complete the payment with the Payments API, or via an alternative payments service provider - if authentication was unsuccessful, the SDK will return an
AuthenticationError
object, which you must handle before proceeding
Note
In rare circumstances, there may be inconsistencies between the SDK output and the authentication status. This can be due to timing differences between the SDK, back end, Access Control Server (ACS), and card scheme during the flow.
To ensure that you receive the accurate authentication status (session status), we recommend that you implement one of the following at the end of every authentication flow:
- perform a GET session request, referencing the session status
- use the
authentication_approved
,authentication_expired
, orauthentication_failed
webhooks to understand the true session status of the flow
1checkout3DS.authenticate(authenticationParameters, context) { result: AuthenticationResult ->2when (result.resultType) {3ResultType.Completed -> {4// Handle authentication result - continue with the payment based on transaction status5val authenticationCompleted: AuthenticationCompleted = (authResult as AuthenticationCompleted)6val sdkTransactionId = authenticationCompleted.sdkTransactionId7val transactionStatus = authenticationCompleted.transactionStatus8}910ResultTypeError -> {11// Handle error (result as AuthenticationError)1213// Handle error based on error type category14val errorType: AuthenticationErrorType = (result as AuthenticationError).errorType1516// Handle error based on fine grained error code or simply log the error17val errorCode: String = (result as AuthenticationError).errorCode18}19}20}
The value of transactionStatus
returned in the AuthenticationResult
object determines whether you can proceed with requesting a payment authorization:
Value | Description | Proceed to payment authorization |
---|---|---|
| Attempt at processing performed | |
| Informational only | |
| Not authenticated or account not verified | |
| Authentication or account verification rejected | |
| Authentication or account verification could not be performed | |
| Authentication verification successful |
Information
You can view a transaction's status from the Payments > Processing > All Payments section of the Dashboard.
With the default SDK implementation, the 3DS challenge screens presented to your customers are displayed with a clean, minimalist UI.
The SDK also provides built-in display and accessibility features:
- support for different screen sizes and orientations
- dynamic text sizing
- support for screen-reading technologies, including VoiceOver on iOS and TalkBack on Android
- support for 37 languages
If you want to customize the screen to match your app's visual design, you can use the SDK's customization options to change the following properties:
- fonts and font colors
- navigation bar and footer background colors
- action button background colors, opacity, shadows, and corner shapes
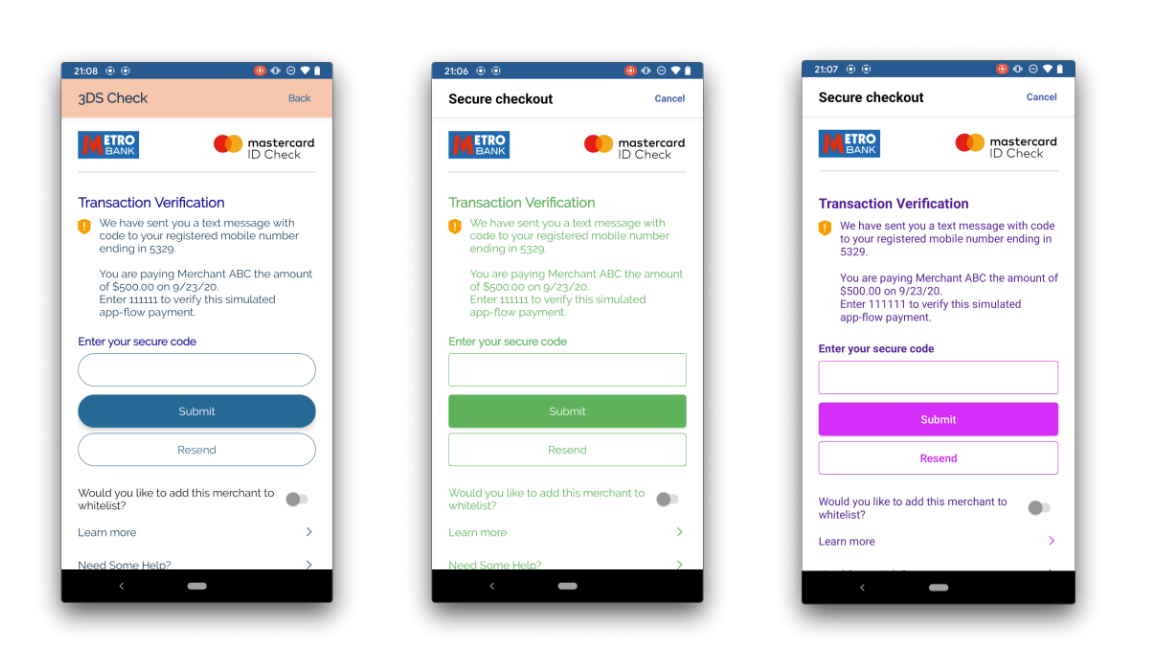
To customize the UI, create a new UICustomization
instance and include it as an argument when you initialize Checkout3DSService
.
The following example demonstrates the customization options available:
1uiCustomization {2all {3textColor = R.color.styleGreenColor4textFont = R.font.raleway5tint = R.color.styleGreenColor6}7}
1uiCustomization {2navigationBar {3backgroundColor = R.color.customStyle1Color14panelElevation = R.dimen.navigationBarElevation156heading {7textColor = R.color.customStyle1Color68text = R.string.navigationBarHeadingText19textFont = R.font.raleway10}1112button {13textColor = R.color.customStyle1Color214text = R.string.navigationBarButtonText115textFont = R.font.raleway16}17}1819informationHeader {20textColor = R.color.customStyle1Color121textFont = R.font.raleway22}2324informationText {25textColor = R.color.customStyle1Color126textFont = R.font.raleway27}2829entryLabel {30textColor = R.color.customStyle1Color131textFont = R.font.raleway32}3334whitelistLabel {35thumbColor = R.color.customStyle1Color236textColor = R.color.customStyle1Color537textFont = R.font.raleway38}3940actionButton {41label {42textColor = R.color.customStyle1Color643textFont = R.font.raleway44}4546background {47backgroundColor = R.color.customStyle1Color148cornerRadius = R.dimen.buttonCornerRadius149bgElevation = R.dimen.buttonElevation150}51}5253alternativeButton {54label {55textColor = R.color.customStyle1Color356textFont = R.font.raleway57}5859background {60backgroundColor = R.color.customStyle1Color461borderColor = R.color.customStyle1Color362borderWidth = R.dimen.buttonBorderWidth163cornerRadius = R.dimen.buttonCornerRadius164bgElevation = R.dimen.buttonElevation165}66}6768entryBox {69input {70textColor = R.color.customStyle1Color171textFont = R.font.raleway72}73background {74backgroundColor = R.color.customStyle1Color675borderColor = R.color.customStyle1Color276borderWidth = R.dimen.buttonBorderWidth177cornerRadius = R.dimen.buttonCornerRadius178bgElevation = R.dimen.buttonElevation179}80}8182entrySelector {83selectorColor = R.color.customStyle1Color28485label {86textColor = R.color.customStyle1Color187textFont = R.font.raleway88}89}9091footer {92expandIndicatorColor = R.color.customStyle1Color29394label {95textColor = R.color.customStyle1Color596textFont = R.font.raleway97}9899text {100textColor = R.color.customStyle1Color5101textFont = R.font.raleway102}103}104}
In accordance with PCI and EMVCo standards, the 3DS SDK incorporates several security approaches. This maintains the integrity of the SDK and ensures your customers' data is secure.
The 3DS SDK is an encrypted binary. This provides increased protection from a number of threats including tampering and reverse engineering. Additionally, the SDK checks:
- if the app was installed from a trusted app store and sends this information to the card issuer
- the environment on which it's running and sends warnings to your app and the card issuer if it detects any threat indicators
For example, the following scenarios would be classed as a threat indicator:
- the device has unauthorized modifications (also referred to as a jailbroken device), which could enable unrestricted access to critical files
- the SDK is running on an emulator
- the SDK source code has been tampered with or its functionality has been modified
- a debugging application is attached
Information
After you initialize the SDK, check the security warnings for any potential threat indicators before you proceed with a transaction.
To keep your customers' data secure and protect the 3DS process, the SDK takes the following precautions with sensitive data:
- only collects sensitive data where it is essential for the authentication process and uses it solely for this purpose
- does not disclose sensitive data to channels outside of the 3D Secure process
- does not hardcode sensitive data into the SDK except where necessary and permitted
- deletes all sensitive data elements once the 3DS transaction is complete
For additional protection, the SDK also:
- secures the UI to prevent access from outside the SDK
- employs run-time data protection techniques to prevent access by unauthorized services
- intercepts and handles any external URL requests contained within 3D Secure HTML – during the 3DS process, the SDK prevents the injection and execution of any JavaScript code by 3D Secure HTML, or any other process outside the 3DS SDK
- securely stores any reference data required for the SDK's operation to prevent unauthorized modifications
- uses EMVCo-approved cryptographic algorithms and methods in all cryptography handled by the 3DS SDK, and employs cryptographically strong random number generation
- uses third-party services to help maintain security
The 3DS SDK communicates with various systems managed by the multiple parties involved in the 3D Secure process. Each of these communication channels is secure and all data exchanged is encrypted.
Communication with the Checkout.com 3DS Server is secured using the latest versions of Transport Layer Security (TLS). The device data passed to the Checkout.com 3DS Server and forwarded to the card scheme’s Directory Server (DS) is transferred in an encrypted format.
Communication with the card issuer’s Access Control Server (ACS) is done over a secure channel that is encrypted end-to-end.
The 3DS SDK also uses Certificate Transparency to ensure end-to-end security and to prevent a man-in-the-middle attack. For this purpose, it checks that all certificates were issued by a trusted Certificate Authority.
Due to the use of Certificate Transparency, the 3DS SDK may not be able to establish a secure connection on devices that are connected to certain SSL Proxy Servers.
In these scenarios, the 3DS SDK will return a connectivity error, which you can communicate to your customer. We recommend that your customer switches to a mobile data connection where available.
We regularly update our 3DS SDK and review our security guidance.
As with our core Standalone authentication product, you can use the 3DS SDK to authenticate a payment and continue to payment authorization with a different payment services provider.
You can also use the 3DS SDK to authenticate with a different provider as part of a custom solution. Contact your account manager or [email protected] to discuss this option.