Get started
Last updated: May 7, 2024
Learn how to embed a pre-built payment user interface in your website to accept payments from your customers. We'll walk you through using our Flow solution, which manages the end-to-end payment experience, including:
- tokenizing sensitive payment details so they never reach your server
- displaying available payment methods
- capturing any additional customer data required
- handling 3D Secure (3DS) authentication and redirection to third-party payment pages
Information
Use the following card details in the interactive demo to perform a test payment:
- Cardholder name: Jordan Smith
- Card number: 4242 4242 4242 4242
- Expiry date: 12/30
- Security code: 100
The customer lands on the checkout page.
You perform a server-side request to create a payment session.
You use the payment session data on the client-side to mount Flow.
Flow displays the available payment methods to the customer. If the customer's chosen payment method requires additional customer data, Flow captures this from them.
When the customer clicks the Pay option, Flow performs a payment request and handles any additional actions. For example, additional actions required for 3D Secure (3DS) authentication, or a third-party payment page redirect.
You receive a webhook notifying you of the payment status.
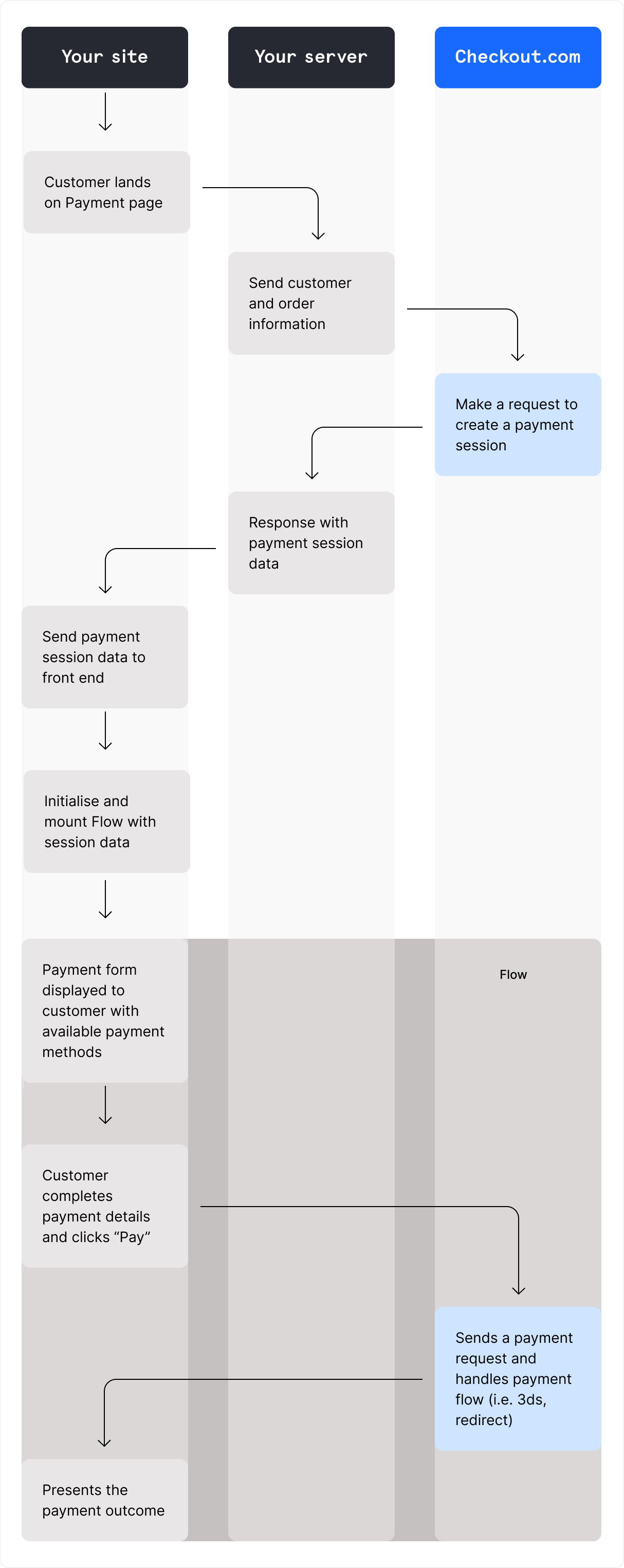
Make sure you have a test account with Checkout.com.
Create a public key and a secret key from the Dashboard, with the following key scopes:
- public key:
payment-sessions:pay
andvault-tokenization
- secret key:
payment-sessions
To receive notifications for the payment events that may be triggered throughout the steps, configure your webhook receiver.
When the customer is ready to pay, you must send a server-side request to securely create a PaymentSession
object. The PaymentSession
contains the information required to handle all steps of the payment flow.
To create a new PaymentSession
, call the following endpoint with your secret key:
post
https://api.checkout.com/payment-sessions
1{2"amount": 1000,3"currency": "GBP",4"reference": "ORD-123A",5"billing": {6"address": {7"country": "GB"8}9},10"customer": {11"name": "Jia Tsang",12"email": "[email protected]"13},14"success_url": "https://example.com/payments/success",15"failure_url": "https://example.com/payments/failure"16}
1{2"id": "ps_2Un6I6lRpIAiIEwQIyxWVnV9CqQ",3"payment_session_token": "YmFzZTY0:eyJpZCI6InBzXzJVbjZJNmxScElBaUlFd1FJeXhXVm5WOUNxUSIsImFtb3VudCI6MTAwMCwibG9jYWxlIjoiZW4tR0IiLCJjdXJyZW5jeSI6IkdCUCIsInBheW1lbnRfbWV0aG9kcyI6W3sidHlwZSI6ImNhcmQiLCJjYXJkX3NjaGVtZXMiOlsiVmlzYSIsIk1hc3RlcmNhcmQiLCJBbWV4Il19XSwicmlzayI6eyJlbmFibGVkIjp0cnVlfSwiX2xpbmtzIjp7InNlbGYiOnsiaHJlZiI6Imh0dHBzOi8vYXBpLnNhbmRib3guY2hlY2tvdXQuY29tL3BheW1lbnQtc2Vzc2lvbnMvcHNfMlVuNkk2bFJwSUFpSUV3UUl5eFdWblY5Q3FRIn19fQ==",4"_links": {5"self": {6"href": "https://api.sandbox.checkout.com/payment-sessions/ps_2Un6I6lRpIAiIEwQIyxWVnV9CqQ"7}8}9}
The payment session response determines which payment methods can be displayed to the customer. The response is determined by:
- the customer's device
- the customer's region
- the values you provide for specific fields in your request
- the payment methods that have been activated for your account – to enable additional payment methods on your account, contact your account manager or [email protected]
Information
Some payment methods may require you to provide additional fields or specific values.
You can install the @checkout.com/checkout-web-components
package via npm:
1npm install @checkout.com/checkout-web-components --save
Alternatively, you can load the package directly via a script:
1<script src="https://checkout-web-components.checkout.com/index.js" />
To remain PCI compliant, you should only ever load the script directly from https://checkout-web-components.checkout.com
. Do not download or host the script yourself, or include it in a bundle.
If you use the template, you must set up your own server.
1<!doctype html>2<html lang="en">3<head>4<meta charset="utf-8" />5<meta name="viewport" content="width=device-width, initial-scale=1.0" />6<title>Checkout Web Components: Flow Component</title>7<link rel="stylesheet" href="./style.css" />8</head>910<body>11<div id="successToast" class="toast success">12<span>The payment was successful</span>13</div>1415<div id="failedToast" class="toast failed">16<span>The payment failed, try again</span>17</div>1819<form>20<div class="container">21<div id="flow-container"></div>22</div>23<span id="error-message"></span>24<span id="successful-payment-message"></span>25</form>2627<script src="https://checkout-web-components.checkout.com/index.js"></script>2829<script src="app.js"></script>30</body>31</html>
The client side will initialize an instance of CheckoutWebComponents
with configuration information and the payment session data retrieved in step 1.
1// Insert your public key here2const publicKey = '{YOUR_PUBLIC_KEY}';34const checkout = await CheckoutWebComponents({5paymentSession,6publicKey,7environment: 'sandbox',8});
You can use the following configuration options to instantiate an instance of CheckoutWebComponents
:
JavaScript key | Description | Required |
---|---|---|
| Your public API key. Your key will be prefixed with | |
| The response from the | |
| Sets the customer's locale. Explicitly setting this value will override the | |
| Sets the environment that the library should point, and send requests to. Use | |
| Allows you to provide custom text translations for a natively supported languages, or add custom text for locales and languages not natively supported by Flow. To find out how to add translations, refer to the Add localization to your Flow integration documentation. | |
| Allows you to customize the visual appearance of the |
You can use CheckoutWebComponents
to create flow
. flow
contains a dynamic list of all of the payment methods available for the payment session you passed into CheckoutWebComponents
.
1const flowComponent = checkout.create('flow');
Mount Flow onto the website using the flow.mount()
method. The method takes a Selector
or an Element
as an argument. For example, #flow-container
or document.getElementById(#flow-container)
, respectively.
1flowComponent.mount('#flow-container');
Note
Embedding Flow within an iframe or onto a Shadow DOM is not supported.
You can use Flow to handle payment methods which require a redirect (asynchronous payments), and those that do not (synchronous payments).
Some payment methods and 3D Secure authentication flows will redirect the customer to a success or failure URL on your website, depending on the outcome of the payment.
A webhook will be sent to your server notifying you of changes to the payment's status. Wait for the webhook before you trigger your order fulfillment process.
Optionally, you can retrieve the payment result from your server, perform a Get payment details
request using the cko-payment-id
in the URL. For example:
1https://example.com/success?cko-payment-id=pay_mbabizu24mvu3mela5njyhpasd
Payment methods that do not require a redirect trigger an onPaymentCompleted
event when the payment is successfully completed. You can use this to notify the customer of the payment status.
A webhook will be sent to your server notifying you of changes to the payment's status. Wait for the webhook before you trigger your order fulfillment process.
1const checkout = await CheckoutWebComponents({2paymentSession,3publicKey,4onPaymentCompleted: async (_self, paymentResponse) => {5// Handle synchronous payments6await handlePayment(paymentResponse.id);7},8});
Optionally, you can retrieve the payment result from your server, perform a Get payment details
request using the cko-payment-id
in the URL. For example:
1https://example.com/success?cko-payment-id=pay_mbabizu24mvu3mela5njyhpasd
Use our test cards to simulate different payment flows, to ensure your integration is set up correctly and behaving as expected.
You can check on the status of a payment from the Payments screen in the Dashboard.