Customize the Frames iOS SDK
Last updated: October 20, 2022
When a customer initiates a payment with the Frames iOS SDK, their payment information is collected via two customizable forms:
- Payment Form: prompts users for their payment card details
- Billing Form: prompts users for their billing information
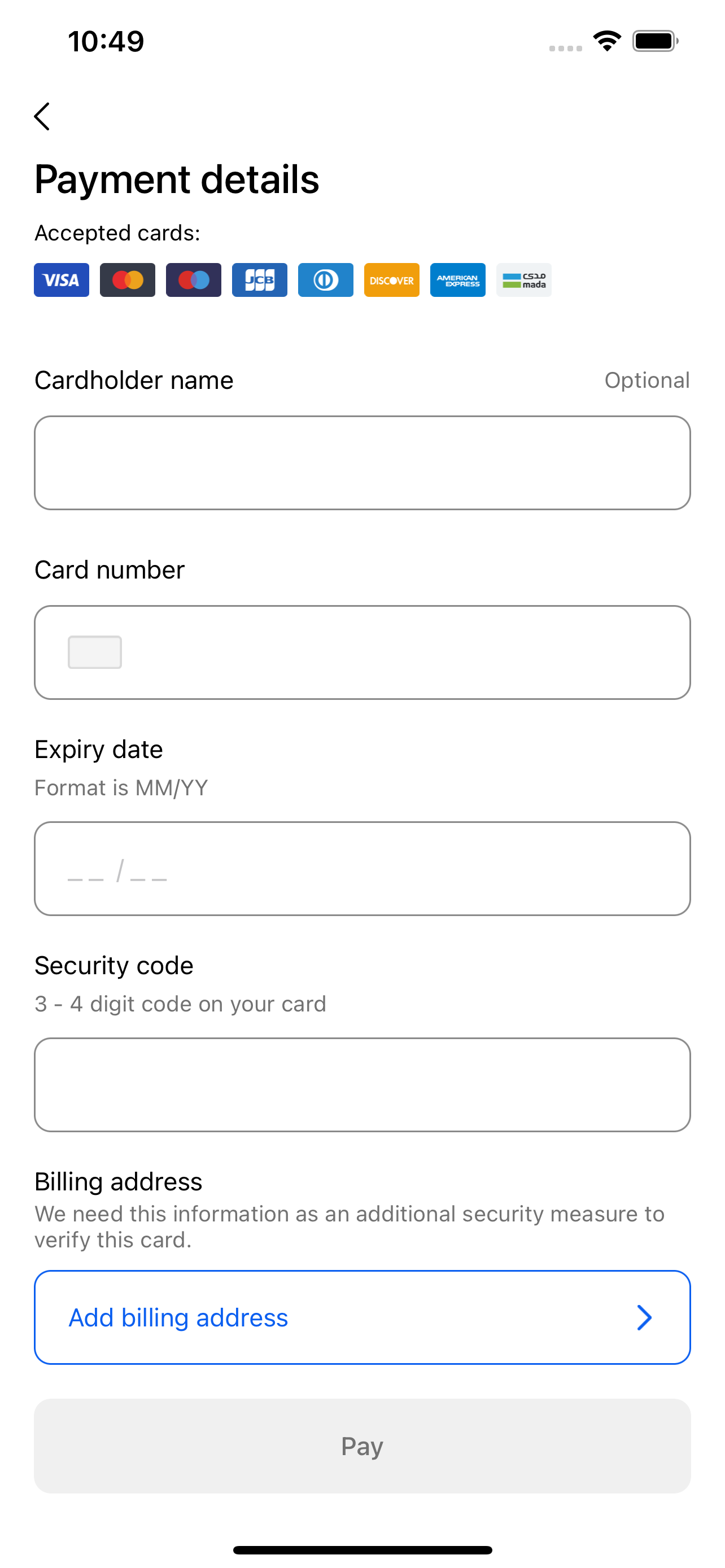
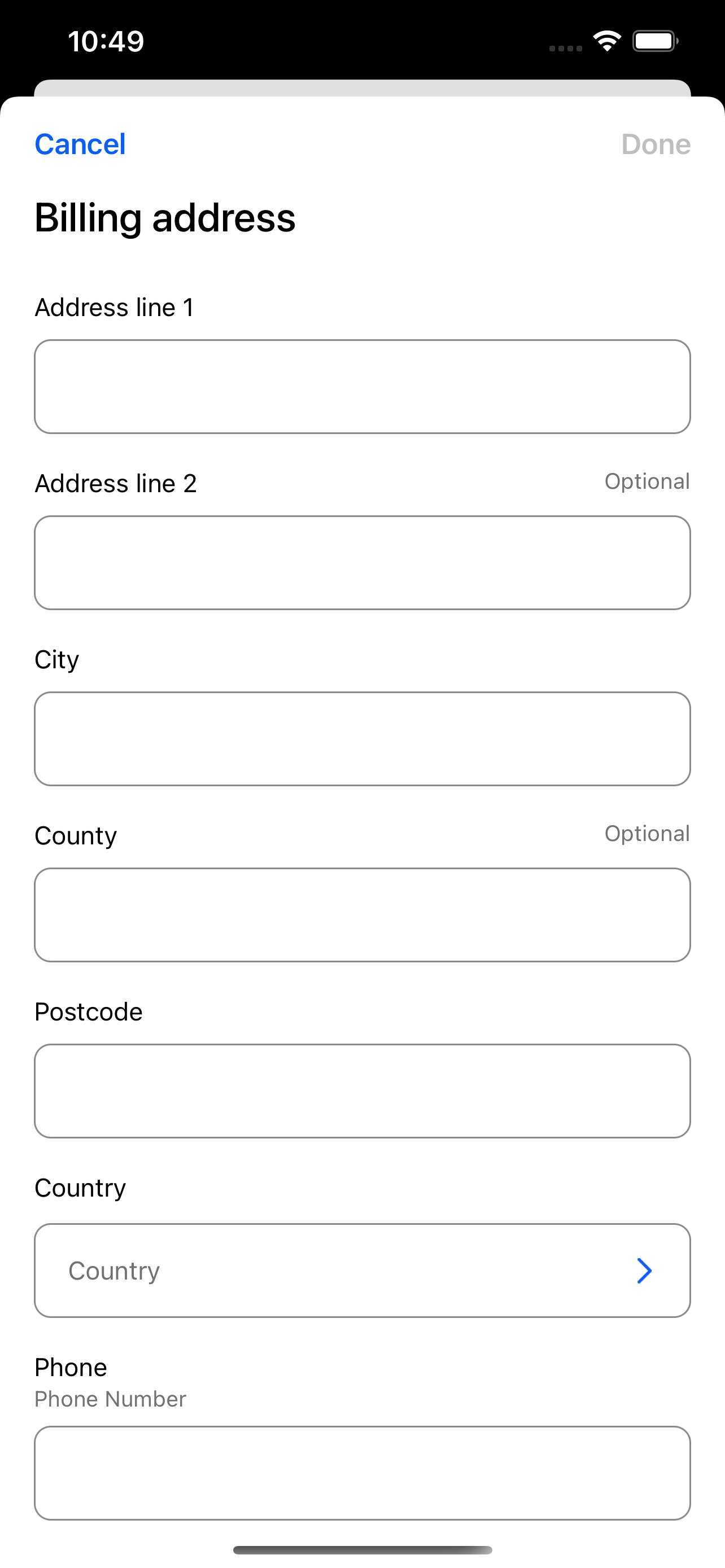
The styling of these forms can be customized through the use of the paymentFormStyle
and billingFormStyle
objects, which are wrapped in the PaymentStyle
wrapper.
1PaymentStyle(paymentFormStyle: YourPaymentStyle(),2billingFormStyle: YourBillingStyle())
You can choose between three different options for customization, depending on how much control you need over the forms' UI design. In order of increasing complexity these are:
The default integration is the quickest way to display the forms required to collect payment information, with no additional customization required.
Localization support for the following languages is built into the default integration:
- Arabic
- Dutch
- English
- French
- German
- Italian
- Romanian
- Spanish
You can also add your own localized strings to add support for additional languages.
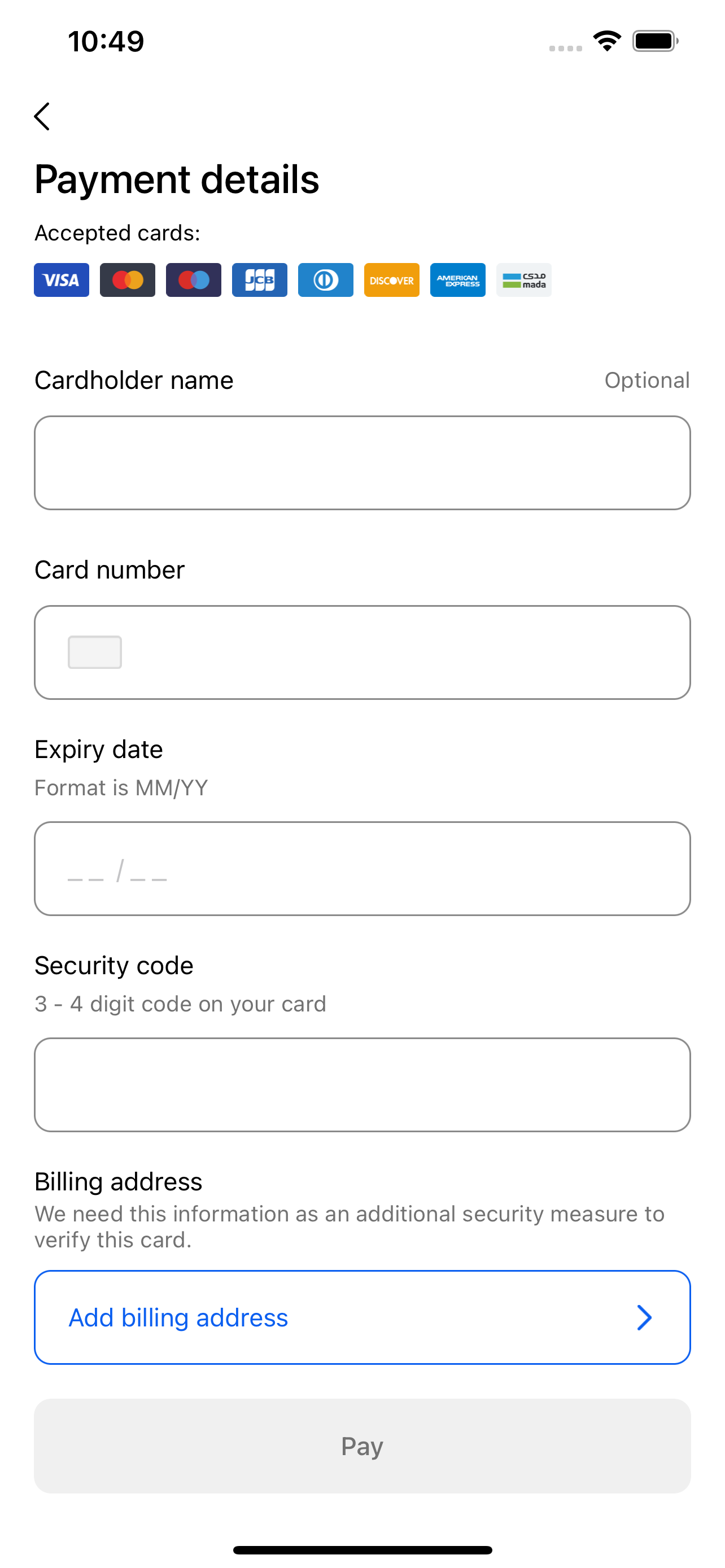
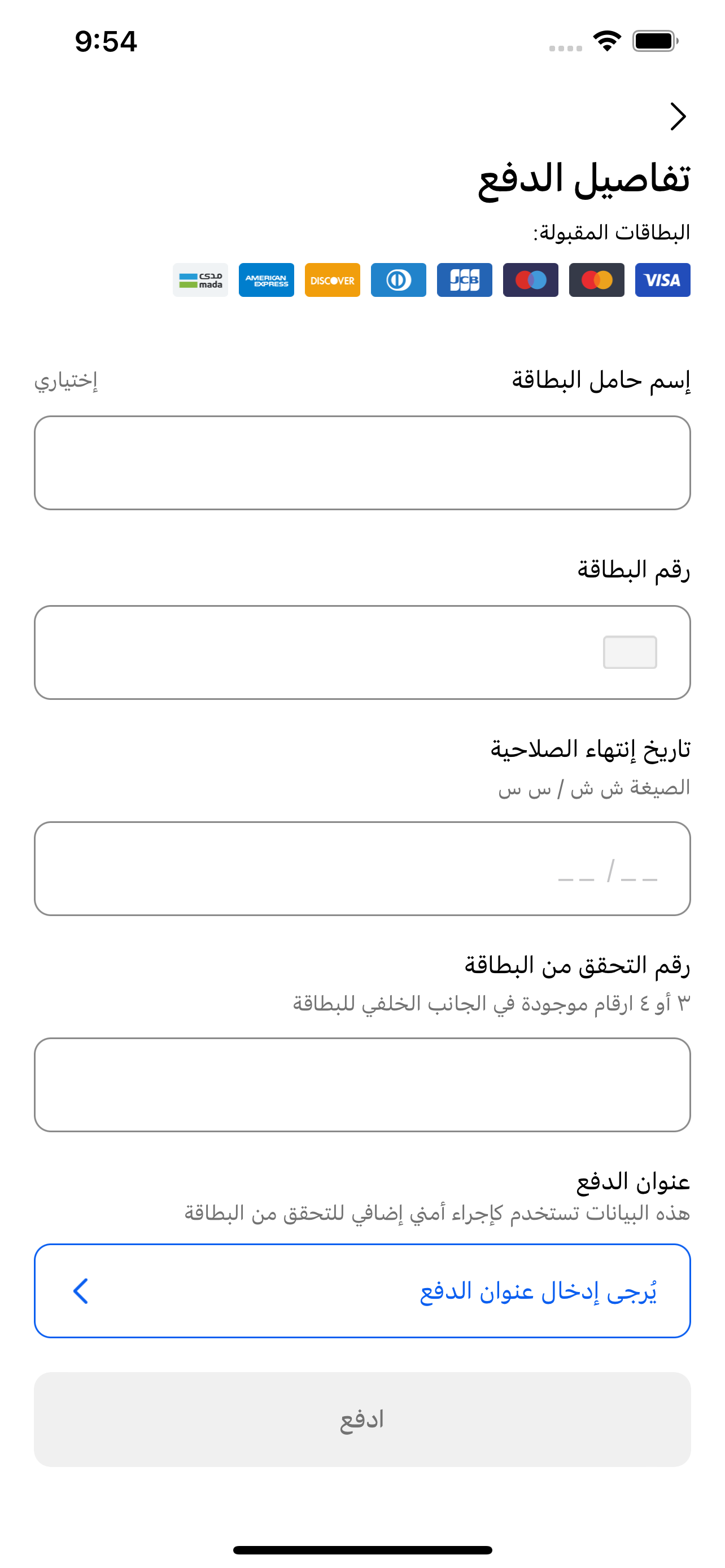
For basic customization, the DefaultPaymentFormStyle
and DefaultBillingFormStyle
objects expose properties that allow you to change the text and colors displayed on the forms.
1// Create a new DefaultPaymentFormStyle object2var paymentFormStyle = DefaultPaymentFormStyle()34// Change the payment form's background color5paymentFormStyle.backgroundColor = UIColor.darkGray67// Change the card number field's placeholder value8paymentFormStyle.expiryDate.textfield.placeholder = "00 / 00"910// Change the payment button text11paymentFormStyle.payButton.text = "Pay £54.63"121314// Create a new DefaultBillingFormStyle object15var billingFormStyle = DefaultBillingFormStyle()1617// Change the billing form header's font color18billingFormStyle.header.headerLabel.textColor = UIColor.darkGray1920// Change the billing form header text21paymentFormStyle.header.headerLabel.text = "Billing info"2223// Wrap the styling in a PaymentStyle wrapper24let paymentStyle = PaymentStyle(paymentFormStyle: paymentFormStyle,25billingFormStyle: billingFormStyle)
See our example project on GitHub.
If you want to customize your forms beyond what is supported by the default integration, you can apply a theme using the Theme
object.
When instantiating a new Theme
object, you must supply the following fields at a minimum:
primaryFontColor
secondaryFontColor
buttonFontColor
errorFontColor
backgroundColor
errorBorderColor
You can then optionally provide additional values to customize other elements including button styling, custom placeholder and error text, and field input controls.
Dynamic font sizing based on the user's device is built-in to the Theme
object.
1// Create a new Theme object2var theme = Theme(primaryFontColor: UIColor(red: 0 / 255, green: 204 / 255, blue: 45 / 255, alpha: 1),3secondaryFontColor: UIColor(red: 177 / 255, green: 177 / 255, blue: 177 / 255, alpha: 1),4buttonFontColor: .green,5errorFontColor: .red,6backgroundColor: UIColor(red: 23 / 255, green: 32 / 255, blue: 30 / 255, alpha: 1),7errorBorderColor: .red)89// Add a border and corner radius around text input fields10theme.textInputBackgroundColor = UIColor(red: 36 / 255.0, green: 48 / 255.0, blue: 45 / 255.0, alpha: 1.0)11theme.textInputBorderRadius = 41213// Add a border around input sections14theme.borderRadius = 41516// Create a new payment form style, only providing custom text values17var paymentFormStyle = theme.buildPaymentForm(18headerView: theme.buildPaymentHeader(title: "Payment details",19subtitle: "Accepting your favorite payment methods"),20addBillingButton: theme.buildAddBillingSectionButton(text: "Add billing details",21isBillingAddressMandatory: false,22titleText: "Billing details"),23billingSummary: theme.buildBillingSummary(buttonText: "Change billing details",24titleText: "Billing details"),25cardNumber: theme.buildPaymentInput(isTextFieldNumericInput: true,26titleText: "Card number",27errorText: "Please enter valid card number"),28expiryDate: theme.buildPaymentInput(textFieldPlaceholder: "__ / __",29isTextFieldNumericInput: false,30titleText: "Expiry date",31errorText: "Please enter valid expiry date"),32securityCode: theme.buildPaymentInput(isTextFieldNumericInput: true,33titleText: "CVV date",34errorText: "Please enter valid security code"),35payButton: theme.buildPayButton(text: "Pay now"))3637// Override a custom property from the resulting payment form style38paymentFormStyle.payButton.disabledTextColor = UIColor.lightGray3940// Create a new billing form style, and modify each field's properties individually41var billingFormStyle = theme.buildBillingForm(42header: theme.buildBillingHeader(title: "Billing information",43cancelButtonTitle: "Cancel",44doneButtonTitle: "Done"),45cells: [.fullName(theme.buildBillingInput(text: "", isNumericInput: false, isMandatory: false, title: "Your name")),46.addressLine1(theme.buildBillingInput(text: "", isNumericInput: false, isMandatory: true, title: "Address")),47.city(theme.buildBillingInput(text: "", isNumericInput: false, isMandatory: true, title: "City")),48.country(theme.buildBillingCountryInput(buttonText: "Select your country", title: "Country")),49.phoneNumber(theme.buildBillingInput(text: "", isNumericInput: true, isMandatory: true, title: "Phone number"))])
See our example project on GitHub.
For complete control over how the various UI elements on your forms look, you can use the fully custom solution.
You can use the properties listed in the structs
below to edit each individual UI component displayed across the forms:
1private enum Constants {2static let mainFontColor = UIColor(red: 0 / 255, green: 204 / 255, blue: 45 / 255, alpha: 1)3static let secondaryFontColor = UIColor(red: 177 / 255, green: 177 / 255, blue: 177 / 255, alpha: 1)4static let errorColor = UIColor.red5static let backgroundColor = UIColor(red: 23 / 255, green: 32 / 255, blue: 30 / 255, alpha: 1)6static let textFieldBackgroundColor = UIColor(red: 36 / 255.0, green: 48 / 255.0, blue: 45 / 255.0, alpha: 1.0)7static let borderRadius: CGFloat = 48static let borderWidth: CGFloat = 19}1011// Main payment form1213struct PaymentFormStyleCustom1: PaymentFormStyle {14var backgroundColor: UIColor = Constants.backgroundColor15var headerView: PaymentHeaderCellStyle = StyleOrganiser.PaymentHeaderViewStyle()16var addBillingSummary: CellButtonStyle? = StyleOrganiser.BillingStartButton()17var editBillingSummary: BillingSummaryViewStyle? = StyleOrganiser.BillingSummaryStyle()18var cardholderInput: CellTextFieldStyle?19var cardNumber: CellTextFieldStyle = StyleOrganiser.CardNumberSection()20var expiryDate: CellTextFieldStyle = StyleOrganiser.ExpiryDateSection()21var securityCode: CellTextFieldStyle? = StyleOrganiser.SecurityNumberSection()22var payButton: ElementButtonStyle = StyleOrganiser.PayButtonFormStyleCustom1()23}2425struct BillingFormStyleCustom1: BillingFormStyle {26var mainBackground: UIColor = Constants.backgroundColor27var header: BillingFormHeaderCellStyle = StyleOrganiser.BillingHeaderViewStyle()28var cells: [BillingFormCell] = [29.fullName(StyleOrganiser.BillingFullNameInput()),30.addressLine1(StyleOrganiser.BillingAddressLine1Input()),31.addressLine2(StyleOrganiser.BillingAddressLine2Input()),32.city(StyleOrganiser.BillingCityInput()),33.state(StyleOrganiser.BillingStateInput()),34.postcode(StyleOrganiser.BillingPostcodeInput()),35.country(StyleOrganiser.BillingCountryInput()),36.phoneNumber(StyleOrganiser.BillingPhoneInput())37]38}3940private enum StyleOrganiser {4142struct PaymentHeaderViewStyle: PaymentHeaderCellStyle {43var shouldHideAcceptedCardsList = true44var backgroundColor: UIColor = Constants.backgroundColor45var headerLabel: ElementStyle? = PaymentHeaderLabel()46var subtitleLabel: ElementStyle? = PaymentHeaderSubtitle()47var schemeIcons: [UIImage?] = []48}4950struct BillingHeaderViewStyle: BillingFormHeaderCellStyle {51var backgroundColor: UIColor = .clear52var headerLabel: ElementStyle = BillingHeaderLabel()53var cancelButton: ElementButtonStyle = CancelButtonStyle()54var doneButton: ElementButtonStyle = DoneButtonStyle()55}5657struct BillingHeaderLabel: ElementStyle {58var textAlignment: NSTextAlignment = .natural59var isHidden = false60var text: String = "Billing address"61var font = UIFont(sfMono: .semibold, size: 24)62var backgroundColor: UIColor = .clear63var textColor: UIColor = Constants.mainFontColor64}6566struct PayButtonFormStyleCustom1: ElementButtonStyle {67var image: UIImage?68var textAlignment: NSTextAlignment = .center69var text: String = "Pay 100$"70var font = UIFont.systemFont(ofSize: 15)71var disabledTextColor: UIColor = Constants.secondaryFontColor72var disabledTintColor: UIColor = Constants.mainFontColor.withAlphaComponent(0.2)73var activeTintColor: UIColor = Constants.mainFontColor74var backgroundColor: UIColor = Constants.mainFontColor75var textColor: UIColor = .white76var normalBorderColor: UIColor = .clear77var focusBorderColor: UIColor = .clear78var errorBorderColor: UIColor = .clear79var imageTintColor: UIColor = .clear80var isHidden = false81var isEnabled = true82var height: Double = 5683var width: Double = 084var cornerRadius: CGFloat = 1085var borderWidth: CGFloat = 086var textLeading: CGFloat = 087}8889struct CancelButtonStyle: ElementButtonStyle {90var textAlignment: NSTextAlignment = .natural91var isEnabled = true92var disabledTextColor: UIColor = Constants.secondaryFontColor93var disabledTintColor: UIColor = .clear94var activeTintColor: UIColor = .clear95var imageTintColor: UIColor = .clear96var normalBorderColor: UIColor = .clear97var focusBorderColor: UIColor = .clear98var errorBorderColor: UIColor = .clear99var image: UIImage?100var textLeading: CGFloat = 0101var cornerRadius: CGFloat = Constants.borderRadius102var borderWidth: CGFloat = Constants.borderWidth103var height: Double = 60104var width: Double = 70105var isHidden = false106var text: String = "Cancel"107var font = UIFont(sfMono: .semibold, size: 17)108var backgroundColor: UIColor = .clear109var textColor: UIColor = Constants.mainFontColor110}111112struct DoneButtonStyle: ElementButtonStyle {113var textAlignment: NSTextAlignment = .natural114var isEnabled = true115var disabledTextColor: UIColor = Constants.secondaryFontColor116var disabledTintColor: UIColor = .clear117var activeTintColor: UIColor = .clear118var imageTintColor: UIColor = .clear119var normalBorderColor: UIColor = .clear120var focusBorderColor: UIColor = .clear121var errorBorderColor: UIColor = .clear122var image: UIImage?123var textLeading: CGFloat = 0124var cornerRadius: CGFloat = Constants.borderRadius125var borderWidth: CGFloat = Constants.borderWidth126var height: Double = 60127var width: Double = 70128var isHidden = false129var text: String = "Done"130var font = UIFont(sfMono: .semibold, size: 17)131var backgroundColor: UIColor = .clear132var textColor: UIColor = Constants.mainFontColor133}134135struct PaymentHeaderLabel: ElementStyle {136var textAlignment: NSTextAlignment = .natural137var isHidden = false138var text: String = "Payment details"139var font = UIFont(size: 24)140var backgroundColor: UIColor = .clear141var textColor: UIColor = Constants.mainFontColor142}143144struct PaymentHeaderSubtitle: ElementStyle {145var textAlignment: NSTextAlignment = .natural146var isHidden = false147var text: String = "Visa, Mastercard and Maestro accepted."148var font = UIFont(size: 12)149var backgroundColor: UIColor = .clear150var textColor: UIColor = Constants.mainFontColor151}152153struct CardNumberSection: CellTextFieldStyle {154var isMandatory = true155var backgroundColor: UIColor = .clear156var textfield: ElementTextFieldStyle = TextFieldStyle()157var title: ElementStyle? = TitleStyle(text: "Card number")158var mandatory: ElementStyle? = MandatoryStyle(text: "")159var hint: ElementStyle?160var error: ElementErrorViewStyle? = ErrorViewStyle(text: "Insert a valid card number")161}162163struct ExpiryDateSection: CellTextFieldStyle {164var textfield: ElementTextFieldStyle = TextFieldStyle(placeholder: "_ _ / _ _")165var isMandatory = true166var backgroundColor: UIColor = .clear167var title: ElementStyle? = TitleStyle(text: "Expiry date")168var mandatory: ElementStyle? = MandatoryStyle(text: "")169var hint: ElementStyle?170var error: ElementErrorViewStyle? = ErrorViewStyle(text: "Insert a valid expiry date")171}172173struct SecurityNumberSection: CellTextFieldStyle {174var textfield: ElementTextFieldStyle = TextFieldStyle()175var isMandatory = true176var backgroundColor: UIColor = .clear177var title: ElementStyle? = TitleStyle(text: "Security code")178var mandatory: ElementStyle? = MandatoryStyle(text: "")179var hint: ElementStyle?180var error: ElementErrorViewStyle? = ErrorViewStyle(text: "Insert a valid security code")181}182183struct TextFieldStyle: ElementTextFieldStyle {184var textAlignment: NSTextAlignment = .natural185var text: String = ""186var isSupportingNumericKeyboard = true187var height: Double = 56188var cornerRadius: CGFloat = Constants.borderRadius189var borderWidth: CGFloat = Constants.borderWidth190var placeholder: String?191var tintColor: UIColor = Constants.mainFontColor192var normalBorderColor: UIColor = .clear193var focusBorderColor: UIColor = .clear194var errorBorderColor: UIColor = Constants.errorColor195var isHidden = false196var font = UIFont(size: 15)197var backgroundColor: UIColor = Constants.textFieldBackgroundColor198var textColor: UIColor = Constants.mainFontColor199}200201struct TitleStyle: ElementStyle {202var textAlignment: NSTextAlignment = .natural203var text: String204var isHidden = false205var font = UIFont(size: 15)206var backgroundColor: UIColor = .clear207var textColor: UIColor = Constants.mainFontColor208}209210struct MandatoryStyle: ElementStyle {211var textAlignment: NSTextAlignment = .natural212var text: String213var isHidden = false214var font = UIFont(size: 13)215var backgroundColor: UIColor = .clear216var textColor: UIColor = Constants.secondaryFontColor217}218219struct SubtitleElementStyle: ElementStyle {220var textAlignment: NSTextAlignment = .natural221var text: String222var textColor: UIColor = Constants.secondaryFontColor223var backgroundColor: UIColor = .clear224var tintColor: UIColor = Constants.mainFontColor225var image: UIImage?226var height: Double = 30227var isHidden = false228var font = UIFont(size: 13)229}230231struct ErrorViewStyle: ElementErrorViewStyle {232var textAlignment: NSTextAlignment = .natural233var text: String234var textColor: UIColor = Constants.errorColor235var backgroundColor: UIColor = .clear236var tintColor: UIColor = Constants.errorColor237var image: UIImage?238var height: Double = 30239var isHidden = true240var font = UIFont(size: 13)241}242243struct BillingStartButton: CellButtonStyle {244var isMandatory = true245var button: ElementButtonStyle = AddBillingDetailsButtonStyle()246var backgroundColor: UIColor = .red// .clear247var title: ElementStyle? = TitleStyle(text: "Billing address")248var mandatory: ElementStyle?249var hint: ElementStyle? = {250var element = SubtitleElementStyle(text: "We need this information as an additional security measure to verify this card.")251element.textColor = Constants.mainFontColor252return element253}()254var error: ElementErrorViewStyle?255}256257struct BillingSummaryStyle: BillingSummaryViewStyle {258var summary: ElementStyle? = BillingSummaryElementStyle(text: "")259var cornerRadius: CGFloat = Constants.borderRadius260var borderWidth: CGFloat = Constants.borderWidth261var separatorLineColor: UIColor = Constants.secondaryFontColor262var borderColor: UIColor = Constants.mainFontColor263var button: ElementButtonStyle = AddBillingDetailsButtonStyle(textLeading: 20, text: "\u{276F} Update Billing Address")264var isMandatory = true265var backgroundColor: UIColor = .clear266var title: ElementStyle? = TitleStyle(text: "Billing address")267var mandatory: ElementStyle?268var hint: ElementStyle? = {269var element = SubtitleElementStyle(text: "We need this information as an additional security measure to verify this card.")270element.textColor = Constants.mainFontColor271return element272}()273var error: ElementErrorViewStyle?274}275276struct AddBillingDetailsButtonStyle: ElementButtonStyle {277var textAlignment: NSTextAlignment = .natural278var isEnabled = true279var disabledTextColor: UIColor = Constants.secondaryFontColor280var disabledTintColor: UIColor = Constants.secondaryFontColor281var activeTintColor: UIColor = Constants.mainFontColor282var imageTintColor: UIColor = .clear283var normalBorderColor: UIColor = .clear284var focusBorderColor: UIColor = .clear285var errorBorderColor: UIColor = .clear286var image: UIImage?287var textLeading: CGFloat = 0288var cornerRadius: CGFloat = 0289var borderWidth: CGFloat = 0290var height: Double = 56291var width: Double = 300292var isHidden = false293var text: String = "\u{276F} Add billing address"294var font = UIFont(sfMono: .semibold, size: 15)295var backgroundColor: UIColor = .clear296var textColor: UIColor = Constants.mainFontColor297}298299struct BillingFullNameInput: CellTextFieldStyle {300var textfield: ElementTextFieldStyle = TextFieldStyle(isSupportingNumericKeyboard: false)301var isMandatory = true302var backgroundColor: UIColor = .clear303var title: ElementStyle? = TitleStyle(text: "Full name")304var mandatory: ElementStyle?305var hint: ElementStyle?306var error: ElementErrorViewStyle?307}308309struct BillingAddressLine1Input: CellTextFieldStyle {310var textfield: ElementTextFieldStyle = TextFieldStyle(isSupportingNumericKeyboard: false)311var isMandatory = true312var backgroundColor: UIColor = .clear313var title: ElementStyle? = TitleStyle(text: "Address line 1")314var mandatory: ElementStyle?315var hint: ElementStyle?316var error: ElementErrorViewStyle?317}318319struct BillingAddressLine2Input: CellTextFieldStyle {320var textfield: ElementTextFieldStyle = TextFieldStyle(isSupportingNumericKeyboard: false)321var isMandatory = false322var backgroundColor: UIColor = .clear323var title: ElementStyle? = TitleStyle(text: "Address line 2")324var mandatory: ElementStyle? = MandatoryStyle(text: "Optional")325var hint: ElementStyle?326var error: ElementErrorViewStyle?327}328329struct BillingCityInput: CellTextFieldStyle {330var textfield: ElementTextFieldStyle = TextFieldStyle(isSupportingNumericKeyboard: false)331var isMandatory = true332var backgroundColor: UIColor = .clear333var title: ElementStyle? = TitleStyle(text: "City")334var mandatory: ElementStyle?335var hint: ElementStyle?336var error: ElementErrorViewStyle?337}338339struct BillingStateInput: CellTextFieldStyle {340var textfield: ElementTextFieldStyle = TextFieldStyle(isSupportingNumericKeyboard: false)341var isMandatory = true342var backgroundColor: UIColor = .clear343var title: ElementStyle? = TitleStyle(text: "State")344var mandatory: ElementStyle?345var hint: ElementStyle?346var error: ElementErrorViewStyle?347}348349struct BillingPostcodeInput: CellTextFieldStyle {350var textfield: ElementTextFieldStyle = TextFieldStyle(isSupportingNumericKeyboard: false)351var isMandatory = true352var backgroundColor: UIColor = .clear353var title: ElementStyle? = TitleStyle(text: "Postcode/Zip")354var mandatory: ElementStyle?355var hint: ElementStyle?356var error: ElementErrorViewStyle?357}358359struct BillingCountryInput: CellButtonStyle {360var button: ElementButtonStyle = BillingCountryButton()361var isMandatory = true362var backgroundColor: UIColor = .clear363var title: ElementStyle? = TitleStyle(text: "Country")364var mandatory: ElementStyle?365var hint: ElementStyle?366var error: ElementErrorViewStyle?367}368369struct BillingPhoneInput: CellTextFieldStyle {370var textfield: ElementTextFieldStyle = TextFieldStyle()371var isMandatory = true372var backgroundColor: UIColor = .clear373var title: ElementStyle? = TitleStyle(text: "Phone number")374var mandatory: ElementStyle?375var hint: ElementStyle? = SubtitleElementStyle(text: "We will only use this to confirm identity if necessary")376var error: ElementErrorViewStyle?377}378379struct BillingSummaryElementStyle: ElementStyle {380var textAlignment: NSTextAlignment = .natural381var isHidden = false382var text: String383var font = UIFont(size: 14)384var backgroundColor: UIColor = .clear385var textColor: UIColor = Constants.secondaryFontColor386}387388struct BillingCountryButton: ElementButtonStyle {389var textAlignment: NSTextAlignment = .natural390var isEnabled = true391var disabledTextColor: UIColor = Constants.secondaryFontColor392var disabledTintColor: UIColor = .clear393var activeTintColor: UIColor = Constants.mainFontColor394var imageTintColor: UIColor = Constants.mainFontColor395var normalBorderColor: UIColor = .clear396var focusBorderColor: UIColor = .clear397var errorBorderColor: UIColor = .clear398var image: UIImage? = UIImage(named: "arrow_green_down")399var textLeading: CGFloat = 20400var cornerRadius: CGFloat = Constants.borderRadius401var borderWidth: CGFloat = 0402var height: Double = 20403var width: Double = 80404var isHidden = false405var text: String = "Please select a country"406var font = UIFont(size: 15)407var backgroundColor: UIColor = Constants.textFieldBackgroundColor408var textColor: UIColor = Constants.secondaryFontColor409}410}
See an example on our GitHub project.